GroveStreams Java & Curl - File Upload Into Stream
This tutorial will walk through the creation of a GroveStreams user account, an organization, and Java code that uploads a local file into a GroveStreams File Stream.
Step 1: Create a Free GroveStreams User Account and Organization
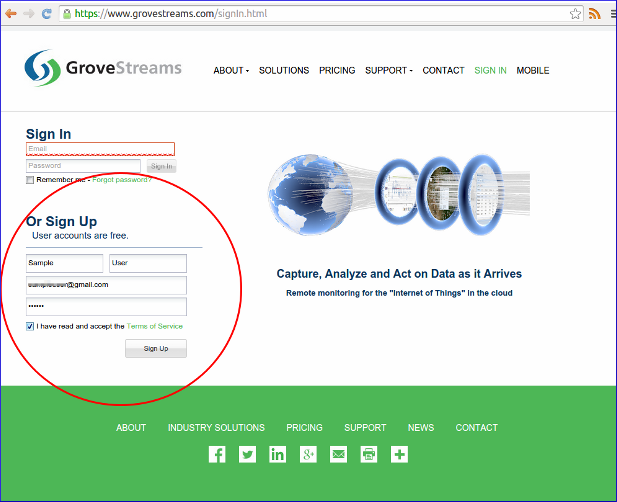
Open a browser and navigate to the GroveStreams registration page and sign up.
Log into GroveStreams after creating your free user account.
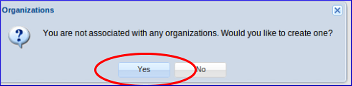
You will be prompted to create an organization. Select Yes.
An organization is a Workspace, typically representing a home, business, or organization. Each organization has its own set of components, streams, dashboards, maps, and other items. An organization allows you to control user and device access to the organization. You are automatically the "owner" and given full access rights when you create an organization. Other users may invite you to their organizations with rights they give you. All of the organizations, you "own" or are a member of, will appear in your GroveStreams start page (the first page that appears when you sign in).
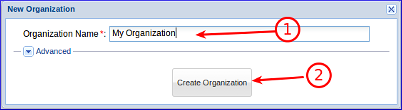
1. Enter a name for the new organization. It can be any name.
2. Click Create Organization.
3. Enter your new organization by clicking on its name.
Step 2: Find your GroveStreams Secret API key for your organization
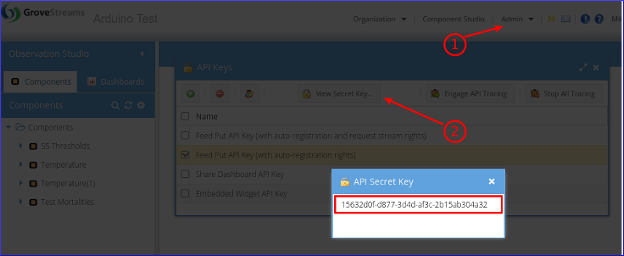
To find your Secret API Key:
1. Select the Admin - API Keys menu option and choose the Feed Put API Key (with auto-registration rights) key
2. Click View Secret Key
3. Select and copy the API Secret Key to the clipboard (Ctrl-c) when you are ready to paste it below.
Step 3: Create the Component File Stream
Files are managed within Component Streams. A component with a file stream will automatically be created by a Feed PUT, the Java code below, if this step is skipped. GroveStreams provides the ability to auto create components and streams using various methods. See our API page for more details. Manually create a Component File Stream within your organization:
1. Enter your organization and right-click on the Components folder and choose New-Component
2. Give your Component a name and ID
3. Right click on Streams and choose Add-Stream-Stream (Most commonly used)
4. Give your stream a name and ID. Set the stream's value type to FILE
5. Save the new Component
Step 4: Code to Upload a File into a Stream (Don't forget to replace the api_key token in
the code below with your Secret API Key!)
Java:
import java.io.File; import java.io.IOException; import java.io.OutputStream; import java.io.OutputStreamWriter; import java.io.PrintWriter; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLConnection; import java.nio.file.Files; public class testSimpleFeedFileUpload { public static void main(String[] args) { try { String compId = "myCompId"; //CHANGE!!! String streamId = "myStreamID"; //CHANGE!!! String api_key = "bd81d152-7101-327a-aaa4-eae5017dc26e"; //CHANGE!!! File binaryFile = new File("GS_logo.jpg"); //CHANGE!!! Add path to local file if needed String urlString = String.format("http://grovestreams.com/api/comp/%s/stream/%s/feed/file?api_key=%s", compId, streamId, api_key); URL url = new URL(urlString); String boundary = Long.toHexString(System.currentTimeMillis()); // Just generate some unique random value. String CRLF = "\r\n"; // Line separator required by multipart/form-data. URLConnection connection = url.openConnection(); connection.setDoOutput(true); connection.setRequestProperty("Content-Type", "multipart/form-data; boundary=" + boundary); OutputStream output = connection.getOutputStream(); PrintWriter writer = new PrintWriter(new OutputStreamWriter(output, "UTF-8"), true); // Send binary file. writer.append("--" + boundary).append(CRLF); writer.append("Content-Disposition: form-data; name=\"binaryFile\"; filename=\"" + binaryFile.getName() + "\"") .append(CRLF); writer.append("Content-Type: " + URLConnection.guessContentTypeFromName(binaryFile.getName())).append(CRLF); writer.append("Content-Transfer-Encoding: binary").append(CRLF); writer.append(CRLF).flush(); Files.copy(binaryFile.toPath(), output); output.flush(); // Important before continuing with writer! writer.append(CRLF).flush(); // CRLF is important! It indicates end of boundary. // End of multipart/form-data. writer.append("--" + boundary + "--").append(CRLF).flush(); // Request is lazily fired whenever you need to obtain information about // response. int responseCode = ((HttpURLConnection) connection).getResponseCode(); System.out.println(responseCode); } catch (IOException e) { e.printStackTrace(); } } }
Curl:
curl -F ‘file=@/home/gs/Pictures/GS_logo.jpg http://grovestreams.com/api/comp/myCompId1/stream/mySreamID/feed/file?api_key=bd81d152-7101-327a-aaa4-eae5017dc26e
1. Change compId to your new component ID
2. Change sreamId to your new file stream ID
3. Change api_key to your organization secret key (see above)
4. Change binaryFile to your local file path and name
Step 5: View the Uploaded File
Double click the file stream within the GroveStreams organization.
The URL to the newly uploaded file will appear. Click the URL to view or download the file. The file can be viewed if the browser supports viewing of the file type. If not, it will automatically download when clicked.
Copy the URl to the clipboard (Ctrl-c) and use it elsewhere such as in GroveStreams dashboards.
IMPORTANT! You may need to append an api_key to the URL if it is used outside an authenticated GroveStreams session.
Post a question on our forum or send us an email and we'll do our best to help you out: support@grovestreams.com
Helpful Links
GroveStreams Help CenterGroveStreams Simple Feed PUT API
GroveStreams Forum